2021 - Bootstrap 5
The responsive ordering classes are now order-first
, order-last
and order-0
- order-5
Demo
2018 - Bootstrap 4
The responsive ordering classes are now order-first
, order-last
and order-0
- order-12
The Bootstrap 4 **push** **pull** classes are now `push-{viewport}-{units}` and `pull-{viewport}-{units}` and the `xs-` infix has been removed. To get the desired 1-3-2 layout on mobile/xs would be: [Bootstrap 4 push pull demo](http://www.codeply.com/go/OmrcmepbUp) (This only works pre 4.0 beta)
Bootstrap 4.1+
Since Bootstrap 4 is flexbox, it's easy to change the order of columns. The cols can be ordered from order-1
to order-12
, responsively such as order-md-12 order-2
(last on md
, 2nd on xs
) relative to the parent .row
.
<div class="container">
<div class="row">
<div class="col-3 col-md-6">
<div class="card card-body">1</div>
</div>
<div class="col-6 col-md-12 order-2 order-md-12">
<div class="card card-body">3</div>
</div>
<div class="col-3 col-md-6 order-3">
<div class="card card-body">2</div>
</div>
</div>
</div>
Demo: Change order using order-*
classes
Desktop (larger screens):
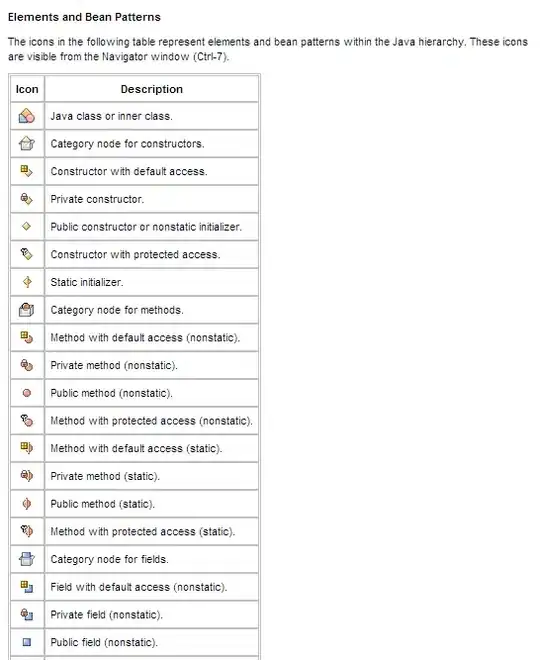
Mobile (smaller screens):
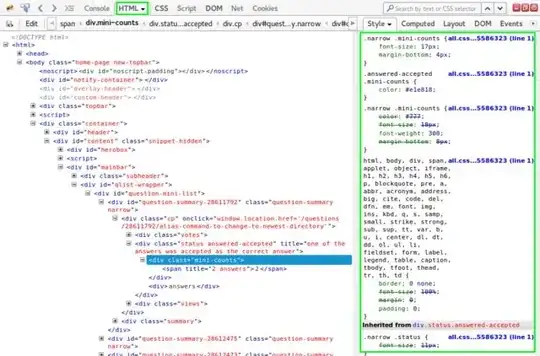
It's also possible to change column order using the flexbox direction utils...
<div class="container">
<div class="row flex-column-reverse flex-md-row">
<div class="col-md-8">
2
</div>
<div class="col-md-4">
1st on mobile
</div>
</div>
</div>
Demo: Bootstrap 4.1 Change Order with Flexbox Direction
Older version demos
demo - alpha 6
demo - beta (3)
See more Bootstrap 4.1+ ordering demos
Related
Column ordering in Bootstrap 4 with push/pull and col-md-12
Bootstrap 4 change order of columns
A-C-B A-B-C