There are multiple options to perform this in windows forms. As an option you can start with customizing RadioButton
and Panel
controls. You can create a new class derived from Panel
and a new class derived from RadioButton
, then override OnPaint
method of those classes and draw the desired presentation.
Here is the result of a sample implementation which I shared in this post:
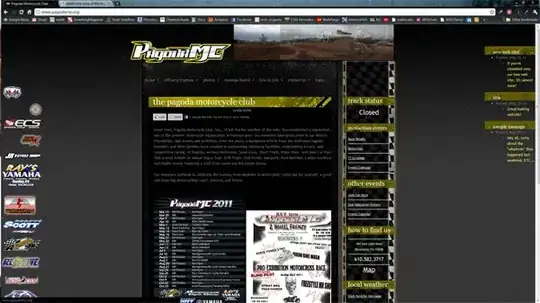
Custom Panel
public class MyPanel : Panel
{
public MyPanel()
{
this.Padding = new Padding(2);
}
protected override void OnPaint(PaintEventArgs e)
{
e.Graphics.SmoothingMode = SmoothingMode.AntiAlias;
using (var path = new GraphicsPath())
{
var d = this.Padding.All;
var r = this.Height - 2 * d;
path.AddArc(d, d, r, r, 90, 180);
path.AddArc(this.Width - r - d, d, r, r, -90, 180);
path.CloseFigure();
using (var pen = new Pen(Color.Silver, d))
e.Graphics.DrawPath(pen, path);
}
}
}
Custom Radio Button
public class MyRadioButton : RadioButton
{
public MyRadioButton()
{
this.Appearance = System.Windows.Forms.Appearance.Button;
this.BackColor = Color.Transparent;
this.TextAlign = ContentAlignment.MiddleCenter;
this.FlatStyle = System.Windows.Forms.FlatStyle.Flat;
this.FlatAppearance.BorderColor = Color.RoyalBlue;
this.FlatAppearance.BorderSize = 2;
}
protected override void OnPaint(PaintEventArgs e)
{
this.OnPaintBackground(e);
using (var path = new GraphicsPath())
{
var c = e.Graphics.ClipBounds;
var r = this.ClientRectangle;
r.Inflate(-FlatAppearance.BorderSize, -FlatAppearance.BorderSize);
path.AddEllipse(r);
e.Graphics.SetClip(path);
base.OnPaint(e);
e.Graphics.SetClip(c);
e.Graphics.SmoothingMode = System.Drawing.Drawing2D.SmoothingMode.AntiAlias;
if (this.Checked)
{
using (var p = new Pen(FlatAppearance.BorderColor,
FlatAppearance.BorderSize))
{
e.Graphics.DrawEllipse(p, r);
}
}
}
}
}
Required usings
using System;
using System.Drawing;
using System.Drawing.Drawing2D;
using System.Windows.Forms;