Image illumination Correction with Perceived Brightness Channel
The value channel of HSV is the maximum of B,G,R values.
So the perceived brightness can be obtained with the following formula.
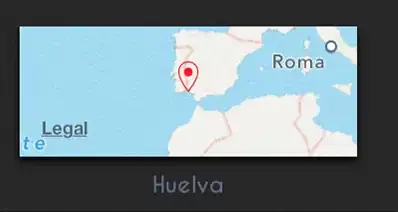
I have applied CLAHE to this channel and It looks good.
I calculate the perceived brightness channel of the image
a - > I change the image into HSV Colour space and I replace the V channel from the image by adding the CLAHE applied perceived brightness channel.
b -> I change the image into LAB Colour space. I replace the L channel from the image by adding the CLAHE applied perceived brightness channel.
Then I again convert the image into BGR format.
The python code for my steps
import cv2
import numpy as np
original = cv2.imread("/content/rqq0M.jpg")
def get_perceive_brightness(img):
float_img = np.float64(img) # unit8 will make overflow
b, g, r = cv2.split(float_img)
float_brightness = np.sqrt(
(0.241 * (r ** 2)) + (0.691 * (g ** 2)) + (0.068 * (b ** 2)))
brightness_channel = np.uint8(np.absolute(float_brightness))
return brightness_channel
perceived_brightness_channel = get_perceive_brightness(original)
clahe = cv2.createCLAHE(clipLimit=3.0, tileGridSize=(8,8))
clahe_applied_perceived_channel = clahe.apply(perceived_brightness_channel)
def hsv_equalizer(img, new_channel):
hsv = cv2.cvtColor(original, cv2.COLOR_BGR2HSV)
h,s,v = cv2.split(hsv)
merged_hsv = cv2.merge((h, s, new_channel))
bgr_img = cv2.cvtColor(merged_hsv, cv2.COLOR_HSV2BGR)
return bgr_img
def lab_equalizer(img, new_channel):
lab = cv2.cvtColor(original, cv2.COLOR_BGR2LAB)
l,a,b = cv2.split(lab)
merged_lab = cv2.merge((new_channel,a,b))
bgr_img = cv2.cvtColor(merged_hsv, cv2.COLOR_LAB2BGR)
return bgr_img
hsv_equalized_img = hsv_equalizer(original,clahe_applied_perceived_channel)
lab_equalized_img = lab_equalizer(original,clahe_applied_perceived_channel)
Output of the hsv_equalized_img
The output of the lab_equlized_img
