The windows only solution from @thingamabobs works also via windll
which comes standard with python (3.11 on surface pro 8, win11),
I converted it into a function:
import tkinter as tk
from ctypes import windll
def maketransparent(w):
# the translated windll part...
# a COLORREF structure is a reverse RGB order int!
# see https://www.pinvoke.net/search.aspx?search=COLORREF&namespace=[All]
# here we chose nearly black so real black (#000000) still shows up
colorkey = 0x00030201
hwnd = w.winfo_id()
wnd_exstyle = windll.user32.GetWindowLongA(hwnd, -20) # GWL_EXSTYLE
new_exstyle = wnd_exstyle | 0x00080000 # WS_EX_LAYERED
windll.user32.SetWindowLongA(hwnd, -20, new_exstyle) # GWL_EXSTYLE
windll.user32.SetLayeredWindowAttributes(hwnd, colorkey, 255, 0x00000001) # LWA_COLORKEY = 0x00000001
win=tk.Tk()
win.geometry('400x200')
win.grid_rowconfigure(0,weight=1)
win.grid_columnconfigure(0,weight=1)
cvs_lower=tk.Canvas(win, background='lightgreen')
cvs_lower.create_rectangle(50, 50, 350, 150, fill='blue')
cvs_lower.grid(row=0, column=0, sticky='nesw')
cvs_upper=tk.Canvas(win, background='#010203') #dont use all black, screws up any black trext on your canvas...
cvs_upper.create_rectangle(325, 25, 375, 175, fill='red')
cvs_upper.grid(row=0, column=0, sticky='nesw')
btn=tk.Button(cvs_upper, text='plop', foreground='#010203', font='Arial 30')
btn.pack(side='right')
win.after(2000, lambda:maketransparent(cvs_upper))
win.mainloop()
So for you, if the draggable canvas is made transparent, its white background will disapear. As in, When chess piece is a white (or black..) filled shape on a canvas with background=colorkey
that back ground will disappear... as is demonstrated with the two canvasses here
Just for completeness, I noticed that any portion of any child of the Canvas cvs_upper
that has the transparency color colorkey
will also be transparent even if the canvas itself may have a drawing on it and is not transparent (e.g. the red bar in the images below).
this is illustrated with a button on the second canvas with its text displayed in the colorkey
color.
before the windows is made transparent we see this:

The canvas is dark (#010203 is v dark!) and the button text is the same color. After a second the upper canvas becomes transparent:
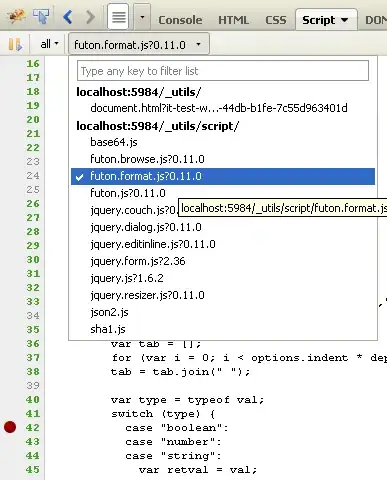
as you can see the canvas and the button text are now transparent, and we see the first canvas below it. We do not see the red bar below the button!