We finally figured a full and correct solution which works in all cases, including stack views, dynamic cells, dynamic number of lines, collection views, animated padding, every character count, and every other situation.
Padding a UILabel
, full solution. Updated for 2023.
It turns out there are three things that must be done.
1. Must call textRect#forBounds with the new smaller size
2. Must override drawText with the new smaller size
3. If a dynamically sized cell, must adjust intrinsicContentSize
In the typical example below, the text unit is in a table view, stack view or similar construction, which gives it a fixed width. In the example we want padding of 60,20,20,24.
Thus, we take the "existing" intrinsicContentSize and actually add 80 to the height.
To repeat ...
You have to literally "get" the height calculated "so far" by the engine, and change that value.
I find that process confusing, but, that is how it works. For me, Apple should expose a call named something like "preliminary height calculation".
Secondly we have to actually use the textRect#forBounds call with our new smaller size.
So in textRect#forBounds we first make the size smaller and then call super.
Alert! You must call super after, not before!
If you carefully investigate all the attempts and discussion on this page, that is the exact problem.
Notice some solutions "seem to usually work". This is indeed the exact reason - confusingly you must "call super afterwards", not before.
If you call super "in the wrong order", it usually works, but fails for certain specific text lengths.
Here is an exact visual example of "incorrectly doing super first":
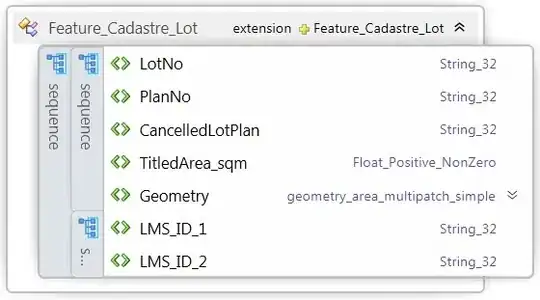
Notice the 60,20,20,24 margins are correct BUT the size calculation is actually wrong, because it was done with the "super first" pattern in textRect#forBounds.
Fixed:
Only now does the textRect#forBounds engine know how to do the calculation properly:
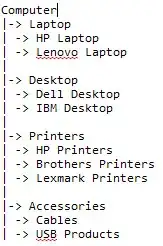
Finally!
Again, in this example the UILabel is being used in the typical situation where width is fixed. So in intrinsicContentSize we have to "add" the overall extra height we want. (You don't need to "add" in any way to the width, that would be meaningless as it is fixed.)
Then in textRect#forBounds you get the bounds "suggested so far" by autolayout, you subtract your margins, and only then call again to the textRect#forBounds engine, that is to say in super, which will give you a result.
Finally and simply in drawText you of course draw in that same smaller box.
Phew!
let UIEI = UIEdgeInsets(top: 60, left: 20, bottom: 20, right: 24) // as desired
override var intrinsicContentSize:CGSize {
numberOfLines = 0 // don't forget!
var s = super.intrinsicContentSize
s.height = s.height + UIEI.top + UIEI.bottom
s.width = s.width + UIEI.left + UIEI.right
return s
}
override func drawText(in rect:CGRect) {
let r = rect.inset(by: UIEI)
super.drawText(in: r)
}
override func textRect(forBounds bounds:CGRect,
limitedToNumberOfLines n:Int) -> CGRect {
let b = bounds
let tr = b.inset(by: UIEI)
let ctr = super.textRect(forBounds: tr, limitedToNumberOfLines: 0)
// that line of code MUST be LAST in this function, NOT first
return ctr
}
Once again. Note that the answers on this and other QA that are "almost" correct suffer the problem in the first image above - the "super is in the wrong place". You must force the size bigger in intrinsicContentSize and then in textRect#forBounds you must first shrink the first-suggestion bounds and then call super.
Summary: you must "call super last" in textRect#forBounds
That's the secret.
Note that you do not need to and should not need to additionally call invalidate, sizeThatFits, needsLayout or any other forcing call. A correct solution should work properly in the normal autolayout draw cycle.